https://school.programmers.co.kr/learn/courses/30/lessons/42748
프로그래머스
SW개발자를 위한 평가, 교육, 채용까지 Total Solution을 제공하는 개발자 성장을 위한 베이스캠프
programmers.co.kr
문제를 따라가며 구현하면 어렵지않은 문제!
배열을 자르고 정렬하고 뽑아내면 됐다..
import java.util.*;
class Solution {
public int[] solution(int[] array, int[][] commands) {
int[] answer = new int[commands.length];
for(int i = 0; i < commands.length; i++){
int startIndex = commands[i][0]-1;
int endIndex = commands[i][1]-1;
int[] splitArray = new int[endIndex - startIndex + 1];
int index = 0;
for(int j = startIndex; j <= endIndex; j++){
splitArray[index++] = array[j];
}
Arrays.sort(splitArray);
answer[i] = splitArray[commands[i][2]-1];
}
return answer;
}
}
여기서 더 최적화하려면 Arrays.copyOfRange() 메서드를 사용할 수 있다.
public static <T> T[] copyOfRange(T[] original, int from, int to)
original: 복사할 원본 배열.
from: 복사 시작 인덱스 (포함).
to: 복사 종료 인덱스 (제외).
리턴값: 지정된 범위에 해당하는 새로운 배열.
import java.util.*;
class Solution {
public int[] solution(int[] array, int[][] commands) {
int[] answer = new int[commands.length];
for(int i = 0; i < commands.length; i++){
int start = commands[i][0] - 1;
int end = commands[i][1];
int k = commands[i][2] - 1;
int[] splitArray = Arrays.copyOfRange(array, start, end);
Arrays.sort(splitArray);
answer[i] = splitArray[k];
}
return answer;
}
}
코드가 훨씬 간결해지고 깔끔해진 것을 확인할 수 있다~!
tmi)
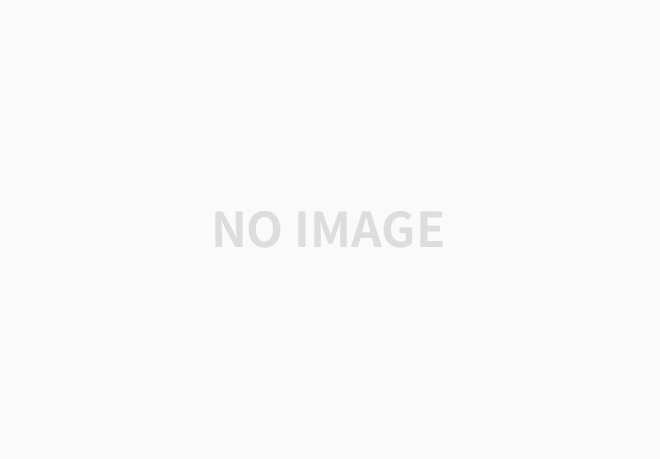
zzz왜 풀 때마다 copyOfRange 가 안 떠오르는 걸까? ^^..
'Algorithm' 카테고리의 다른 글
[Algorithm] 프로그래머스_완주하지 못한 선수(JAVA) (0) | 2024.12.13 |
---|---|
[Algorithm] 프로그래머스_네트워크(JAVA) (0) | 2024.12.11 |
[Algorithm] 프로그래머스_올바른 괄호(JAVA) (3) | 2024.12.10 |
[Algorithm] 프로그래머스_타겟넘버(JAVA) (3) | 2024.12.10 |
[Algorithm] 프로그래머스_특이한 정렬(JAVA), 우선순위 큐, Comparator (6) | 2024.12.05 |